PHP is a multi-dimensional language that can create anything you want. Here is another example of PHP that creates an Online Chess Board Design with Chess Pieces.
Chess Board Design
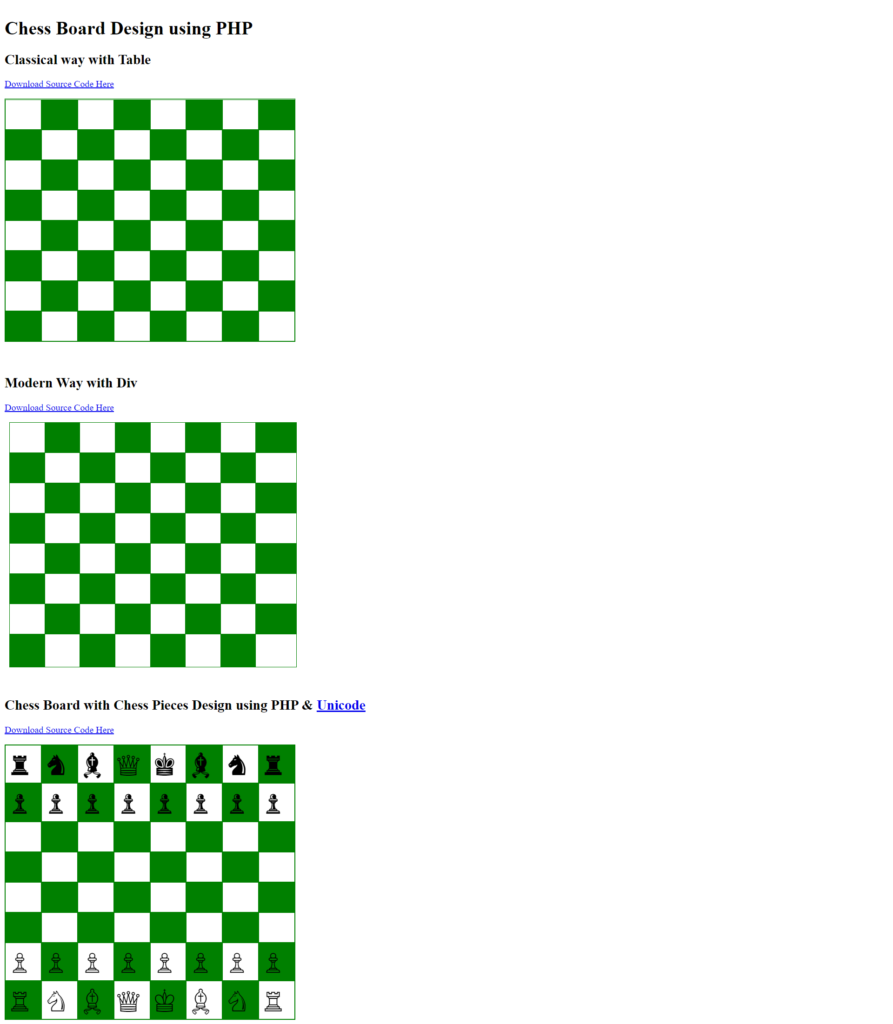
Classical way with Table
<?php
$row=$total=$col=0;
for($row=1;$row<=8;$row++)
{
echo "<tr>";
for($col=1;$col<=8;$col++)
{
$total=$row+$col;
if($total%2==0) {
echo "<td height='50px' width='50px' bgcolor='#FFFFFF'></td>";
}
else{
echo "<td height='50px' width='50px' bgcolor='green'></td>";
}
}
echo "</tr>";
}
?>
Modern Way with Div
<?php
$row=$total=$col=0;
for($row=1;$row<=8;$row++)
{
echo "<div id='line'>";
for($col=1;$col<=8;$col++)
{
$total=$row+$col;
if($total%2==0)
{
echo "<div id='box' class='white'></div>";
}
else
{
echo "<div id='box' class='black'></div>";
}
}
echo "</div> ";
}
?>
Chess Board with Chess Pieces Design using PHP & Unicode
<?php
$row=$total=$col=0;
for($row=1;$row<=8;$row++)
{
echo "<tr>";
if($row==8){
echo "
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♖</div></td>
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♘</div></td>
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♗</div></td>
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♕</div></td>
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♔</div></td>
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♗</div></td>
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♘</div></td>
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♖</div></td>
";
}
elseif($row==1){
echo "
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♜</div></td>
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♞</div></td>
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♝</div></td>
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♕</div></td>
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♚</div></td>
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♝</div></td>
<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♞</div></td>
<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♜</div></td>
";
}
else{
for($col=1;$col<=8;$col++)
{
$total=$row+$col;
if($row==7){
if($total%2==0)
{
echo "<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♙</div></td>";
}
else
{
echo "<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♙</div></td>";
}
}
elseif($row==2){
if($total%2==0)
{
echo "<td height='50px' width='50px' bgcolor='#FFFFFF'><div class='chess-piece'>♟</div></td>";
}
else
{
echo "<td height='50px' width='50px' bgcolor='green'><div class='chess-piece'>♟</div></td>";
}
}
else{
if($total%2==0)
{
echo "<td height='50px' width='50px' bgcolor='#FFFFFF'></td>";
}
else
{
echo "<td height='50px' width='50px' bgcolor='green'></td>";
}
}
}
}
echo "</tr>";
}
?>
Additional Resource: (CSS)
.table{
width: 500px;
padding: 1px;
border:1px solid darkgreen;
}
body{
margin: 20 20 auto;
}
#box{
height:50px;
width:12.5%;
border:1px solid green;
padding:3px;
display:inline-block;
margin-right: -10px;
}
.black{
background:green;
}
.white{
background:white;
}
#line{
width:500px;
text-align: center;
margin-bottom: -10px;
}
#board{
width:500px;
height:100%;
}
.chess-piece{
font-size: 48px;
}